How to Open A WordPress Functions File Using A Hook
If you want to write your own WordPress child theme, or even your own theme, you are most likely going to be getting into the nitty gritty of the functions.php file.
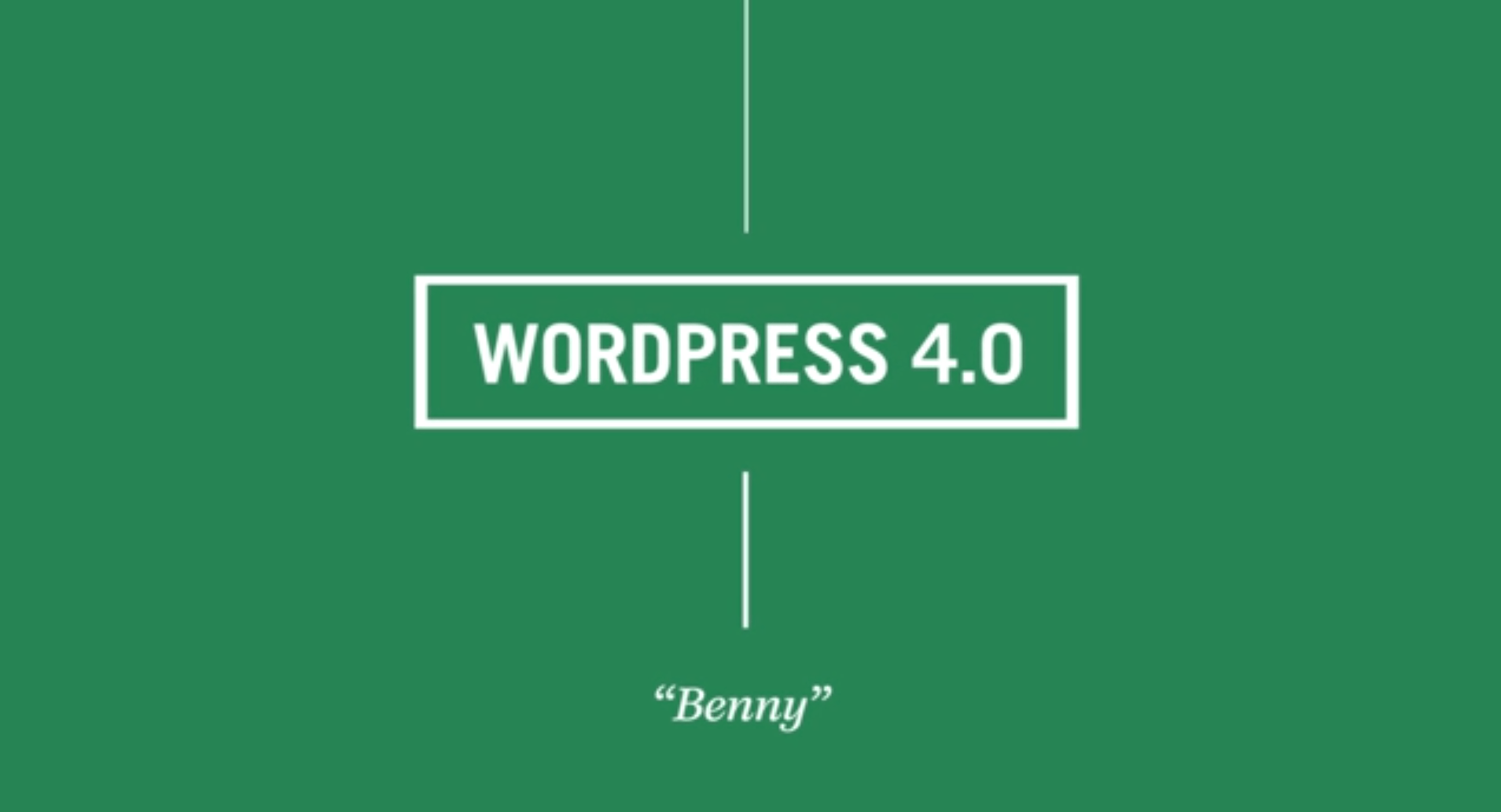
If you want to write your own WordPress child theme, or even your own theme, you are most likely going to be getting into the nitty gritty of the functions.php file.
When you work in this file, you are essentially writing up a series of instructions for WordPress to deploy, in a pre-set order.
A smart way to work in this environment is to set your basic customizations inside a single function of their own, and then trigger that function to fire in a specific order within the WordPress code. How does WordPress know where to to fire your code? Because you tell your function to make use of a WordPress hook.
Hooks are areas within the WordPress code that allow template developers, people like you and me, to take our great customizations and inject them into specific areas of the overall WordPress software. Pretty cool.
Each hook name is a place identifying an area of the WordPress code. Maybe it is helpful to think of it not as a place in the code – but instead as a place in time during the execution of the code? Either way, your WordPress code is a linear set of instructions like this:
A ---> B ---> C ---> D
WordPress does A, then does B, then does C, then finishes with D.
Here’s how that would look with hooks in place:
A(hook1) ---> B(hook2) ---> C(hook3) ---> D(hook4)
So, in this simplified example, if you wanted to add some code that should execute after B, you would use the hook hook2
. Simple? And yes, there is a whole list of WordPress action hooks to look over so you don’t have to guess!
OK, well, a hook that can help you organize your customizations within your functions file is ‘after_theme_setup
‘.
Note: This action hook is called after the page loads.
Here’s how:
- Open your function file with
<?php
Note: no spaces or comments are allowed before the<?php
declaration - To start, use add_action to tell WordPress to use your new function. i.e.
add_action( 'after_setup_theme', 'your_child_theme_setup' );
- Create the function that will hold your instructions, i.e.
your_child_theme_setup() {
- Add your instructions inside your new function, i.e.
add_theme_support( 'post-thumbnails' );
add_theme_support( 'custom-background');
register_nav_menu( 'primary', __( 'Navigation Menu', 'myTheme' ) );
define( 'HEADER_IMAGE_HEIGHT', apply_filters( 'myTheme_header_image_height', 198 ) ); - Close your function with the right french brace “}”
That’s for vanilla WordPress.
If you are using Genesis, there are two ways to go about this. The first way is what is used in many Genesis child themes:
Add this to the top of your functions file (after <?php
)
require_once(TEMPLATEPATH.'/lib/init.php');
This basically retrieves and executes code from the functions file of the parent Genesis core theme.
OK. Then there is this second way, which I like better:
Add this to the top of your functions file (after <?php
)
add_action( 'genesis_setup', 'your_child_theme_setup', 11 );
function your_child_theme_setup() { ... your code}
I prefer this second method (for Genesis) because, just like after_theme_setup
, it places your instructions into a bundle and executes them in a pre-set ordering of actions (after the page loads).
What kind of code can you place inside the genesis_setup
or after_setup_theme
? You can register any theme support functions, add custom filters, and/or actions inside your_child_theme_setup. You would not want to use wp_enqueue_style
functions within your after_theme_setup
simply because there are other more appropriate action hooks for it, like wp_enqueue_scripts
.
Altogether: